3 AM: How to handle REST API calls in flutter like a PRO!!
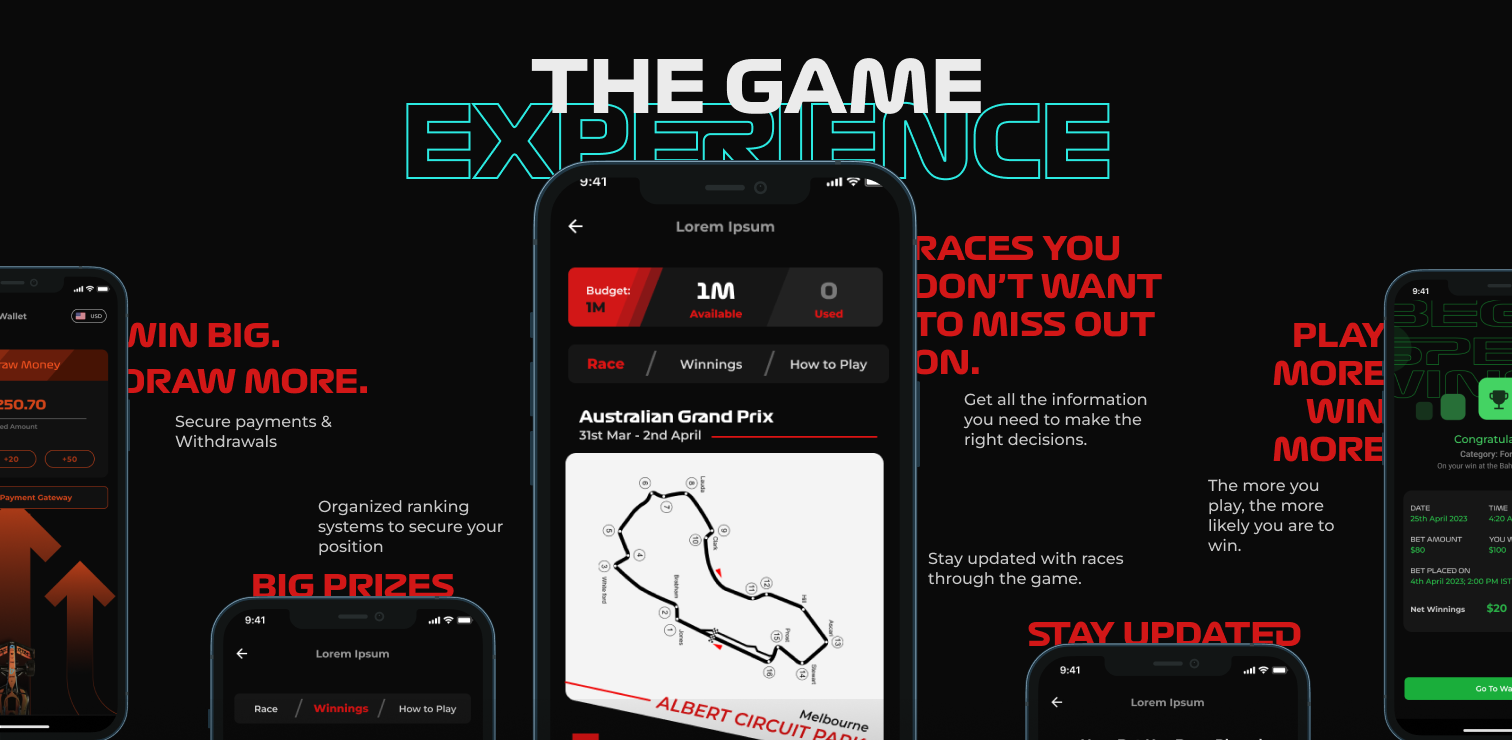
It was 3 AM on a Wednesday. My desk looked like a mess of caffeine—empty Red Bull cans, a forgotten half-eaten sandwich, and at least three coffee mugs in various states of emptiness.
Not ideal.
"It worked perfectly with the test data!" my lead developer groaned for the fifth time, scrolling through our product listing page. Each swipe made our beautiful UI stutter like a teenager asking someone to prom. Not exactly the smooth experience we were promising users.
When Your Fix Makes Things Worse (A Story as Old as Code)
Let me back up a bit. Our Flutter app relies heavily on REST API calls with JSON responses that would make even the most data-loving developer say "Maybe we should make this simpler?" During development with fake data, everything worked fine, but connecting to our real backend was where things went wrong.
The first fix was obvious—move the heavy work to isolates. For non-Flutter folks, isolates are Dart's way of saying "hey, do this hard work somewhere else so my UI thread can focus on looking pretty."
Feeling pretty smart about this obvious fix, we tried it and ran the app again.
It was worse. Not just slightly worse—it was "maybe we should just make a calendar app instead" worse.
After some testing and a brief moment of panic, we found the problem: creating and killing isolates for each API call was like hiring movers to move a single pencil across your desk, then firing them, then hiring them again to move an eraser. The setup cost was killing us.
Why App Speed Matters (Or: Why Users Leave)
At this point, I did what any responsible CEO/CTO would do at 3:30 AM: I started reading articles on how users feel about slow apps. Listen, we all deal with stress differently.
Here's what I learned:
- 0-100ms: Users think it's instant
- 100-300ms: There's a delay, but it's fine
- 300-1000ms: "Is this app working?"
- 1000ms+: Opens a different app
But here's the really interesting part—uneven performance is worse than consistently okay performance. A UI that sometimes freezes makes users start to worry about using certain features because they expect them to lag.
It's like that one squeaky floorboard in your home. Not a big deal really, but you find yourself walking weird paths to avoid stepping on it at 2 AM.
The 4:37 AM Lightbulb Moment
I was staring blankly at my screen, thinking about changing careers to something less technical—like professional dolphin trainer—when it hit me.
"What if," I mumbled to my lead developer (who was face-down on his keyboard, possibly asleep), "we created a pool of isolates when the app starts and reused them?"
He lifted his head, keyboard keys marked on his forehead. "You mean like... thread pooling but for isolates?"
"Exactly! A pool party, but for isolates. And way less fun."
We drew it on our whiteboard (which was actually just a wall we painted with whiteboard paint, and trust me—landlords LOVE that during inspections):
- Create a small group of isolates when the app starts
- When work comes in, it gets in line (newest work first)
- Free isolates grab work from the line like it's pizza at a developer meetup
- If all isolates are busy, new work waits its turn
- If an isolate takes too long, we replace it
- If an isolate crashes, we bring in a new one—like a backup player in sports
The Fix That Saved My Hair
By 7 AM, driven by the fear of looking bad, we had a working test version. We called it the "Isolate Pool Manager" because we're engineers, not marketing people, and naming things creatively would break some unwritten developer rule.
The code wasn't pretty. There were "fix later" notes all over the code, and at least one comment that just said "FIXME: What is even happening here?" But it worked.
Getting the main thread and worker isolates to talk to each other was tricky. By "tricky," I mean three more hours of fixing why messages weren't being passed correctly. Turns out isolates are very picky about how you talk to them—kind of like trying to explain to my grandmother why she doesn't need to yell at her smartphone.
Results That Made Me Wonder Why We Didn't Do This Sooner
When we finally got it working right, the difference was so big I actually thought we'd broken something else. Scrolling was smooth. Pages loaded without the UI freezing. I could quickly tap between tabs without the app showing me the spinning wheel of "please wait while I think about life."
The most satisfying moment was watching our designer scroll through a product list with hundreds of items, waiting for the stuttering that never came. His disappointed face when he couldn't find something to complain about was worth every debugging hour.
Two Weeks After Launch: The Hidden Hero
Fast forward to today—two weeks after launch—and our Isolate Pool Manager has been handling thousands of API requests daily without problems. It's like that one team member who never calls in sick, never complains about the coffee, and somehow makes everyone else look better.
Here's what this whole mess taught me:
- The most obvious fix is almost never the best one. If it were, programming would just be copying and pasting from the internet (I mean, it sometimes is, but don't tell anyone I said that).
- Test first, code later. Without testing, we would've shipped an app that ran worse than if we'd done nothing at all. That's a special kind of failure.
- Think bigger picture. Creating a system to handle resources over time is almost always better than creating and destroying resources for each task. This applies to dating too, but that's a different blog post.
- Fixing things before launch matters. Finding these issues two weeks after launch would've been like trying to change a car's engine while driving on the highway.
- Users don't care about your clever code. They just want the app to feel good. The difference between a good and great app often comes down to how smooth it feels, even if users can't explain why.
How It Actually Works (The Simple Version)
Our solution is pretty straightforward to understand, if not to build:
When the app starts, we create a few isolates (usually 3-4) that stay around for the app's entire life. They're like the app's dedicated data processing team, waiting for work.
Each API request becomes a work item and goes into a queue. A dedicated process watches this queue and gives work to any isolate that's free, like an efficient office manager.
If all isolates are busy, new requests wait their turn. This prevents overloading the system while keeping everything moving.
Each request has a time limit because sometimes isolates get stuck. If a request takes too long, we kill and restart the isolate, keeping the system running smoothly.
We also added monitoring—if an isolate crashes (and they do, at the worst possible times), our system automatically creates a replacement. It's like having a backup ready when the main player gets injured.
What Users Actually Notice
The technical improvements turned into real benefits that users notice (even if they don't know why):
- Scrolling so smooth you could spread it on toast
- UI elements that appear right away while data loads in the background
- Better battery life because we're not wasting resources
- More reliable app because the system fixes itself when things go wrong
From a business view, these improvements matter a lot. Users decide if an app is good in the first few seconds, often before seeing any actual content. Performance problems can make users quit faster than you can say "but our features are amazing!"
Conclusion: Sometimes Boring Wins
It's been just over two weeks since we launched, and I still sometimes open the app just to scroll through lists smoothly, like some kind of weird performance-obsessed person.
Those late nights before the launch were absolutely worth it. There's something really satisfying about fixing a technical problem that makes the whole product better—even if users never say "Wow, your isolate pool management is AMAZING!"
Sometimes the best features are the ones nobody notices because they just work. Kind of like good plumbing—nobody writes reviews about how well the toilet flushed, but they definitely leave one-star reviews when it doesn't.
As both the technical and business leader of our company, I've learned that great products aren't built on flashy features alone. They're built on foundations of speed and reliability that often go unseen but are always felt.
Now if you'll excuse me, I need to go fix that one animation that's off by 2 pixels. My designer has been staring at me for 20 minutes straight and I'm starting to get worried.
Written by our founder based on our pre-launch work. Our app went live just 15 days ago, and despite my team telling me to stop checking app store reviews all the time, I'm excited about how users like the smooth experience.